Functions#
In this “chapter” I will attempt to run through some required function knowledge for calculus.
What is a function?#
The natural world is full of relationships between quantities that change. When we see these relationships, it is natural for us to ask “If I know one quantity, can I then determine the other?” This establishes the idea of an input quantity, or independent variable, and a corresponding output quantity, or dependent variable. From this we get the notion of a functional relationship in which the output can be determined from the input.
For some quantities, like height and age, there are certainly relationships between these quantities. Given a specific person and any age, it is easy enough to determine their height, but if we tried to reverse that relationship and determine height from a given age, that would be problematic, since most people maintain the same height for many years.
Definition of a Function
A function is rule for a relationship between an input, or independent, quantity and an output, or dependent, quantity in which each input value uniquely determines one output value. We say “the output is a function of the input.”
Following are some things to consider: * In the height and age example above, is height a function of age? Is age a function of height? * In the height and age example above, it would be correct to say that height is a function of age, since each age uniquely determines a height. For example, on my 18th birthday, I had exactly one height of 69 inches. * However, age is not a function of height, since one height input might correspond with more than one output age. For example, for an input height of 70 inches, there is more than one output of age since I was 70 inches at the age of 20 and 21.
Function Notation#
To simplify writing out expressions and equations involving functions, a simplified notation is often used. We also use descriptive variables to help us remember the meaning of the quantities in the problem.
Rather than write “height is a function of age,” we could use the descriptive variable h to represent height and we could use the descriptive variable a to represent age.
Example
“Height is a function of age”. If we name the function
Remember we can use any variable to name the function; the notation
The notation output =
defines a function name . This would be read “output is of input”.
Example
A function
Solution
When we read
Table of Values#
Functions can be represented in many ways: Words (as we did in the last few examples), tables of values, graphs, or formulas. Represented as a table, we are presented with a list of input and output values.
This table represents the age of children in years and their corresponding heights. While some tables show all the information we know about a function, this particular table represents just some of the data available for height and ages of children.
Solving and Evaluating Functions#
When we work with functions, there are two typical things we do: evaluate and solve. Evaluating a function is what we do when we know an input, and use the function to determine the corresponding output. Evaluating will always produce one result, since each input of a function corresponds to exactly one output.
Solving equations involving a function is what we do when we know an output, and use the function to determine the inputs that would produce that output. Solving a function could produce more than one solution, since different inputs can produce the same output.
Example
Using the table shown, where
1 | 8 |
2 | 6 |
3 | 7 |
4 | 6 |
5 | 8 |
Table of values for
Evaluate
.Solve
.
Solution
For (a) it is important to realize we want to input
Evaluate
: Evaluating (read: “ g of 3” ) means that we need to determine the output value, , of the function given the input value of . Looking at the table, we see the output corresponding to is , allowing us to conclude ..Solve
: Solving means we need to determine what input values, , produce an output value of . Looking at the table we see there are two solutions: and . When we input into the function , our output is . When we input into the function , our output is also .
Functions and Graphs#
Oftentimes a graph of a relationship can be used to define a function. By convention, graphs are typically created with the input quantity along the horizontal axis and the output quantity along the vertical.
[4]:
import matplotlib.pyplot as plt
import numpy as np
x = np.linspace(-5, 5, 400)
y = x**2
plt.figure()
plt.plot(x, y)
plt.title('Parabola')
plt.xlabel('x')
plt.ylabel('y = x^2')
plt.grid()
plt.axhline(0, color='black', linewidth=0.8) # Add horizontal axis
plt.axvline(0, color='black', linewidth=0.8) # Add vertical axis
plt.show()
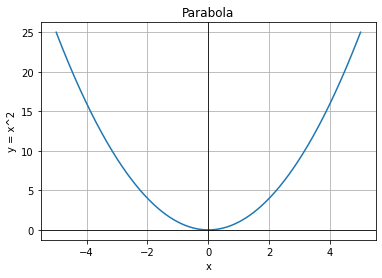
[5]:
theta = np.linspace(0, 2*np.pi, 400)
r = 2
x = r * np.cos(theta)
y = r * np.sin(theta)
plt.figure()
plt.plot(x, y)
plt.title('Circle')
plt.xlabel('x')
plt.ylabel('y')
plt.grid()
plt.axhline(0, color='black', linewidth=0.8) # Add horizontal axis
plt.axvline(0, color='black', linewidth=0.8) # Add vertical axis
plt.show()
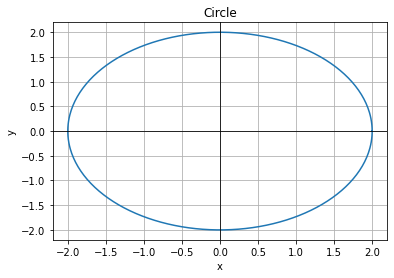
Looking at the two graphs above, the first define a function
The vertical line test is a handy way to think about whether a graph defines the vertical output as a function of the horizontal input. Imagine drawing vertical lines through the graph. If any vertical line would cross the graph more than once, then the graph does not define only one vertical output for each horizontal input.
Evaluating a function using a graph requires taking the given input and using the graph to look up the corresponding output. Solving a function equation using a graph requires taking the given output and looking on the graph to determine the corresponding input.
[14]:
import numpy as np
import matplotlib.pyplot as plt
# Define the equation
def equation(x):
return (x - 1)**2
# Generate x values
x = np.linspace(-1.23606797749979,3.23606797749979, 400)
# Calculate y values using the equation
y = equation(x)
# Set up the figure and plot
plt.figure()
plt.plot(x, y, label='$y = (x - 1)^2$')
plt.title('Plot of $y = (x - 1)^2$')
plt.xlabel('x')
plt.ylabel('y')
plt.grid(True, linestyle='--', linewidth=0.8)
plt.axhline(0, color='black', linewidth=0.8)
plt.axvline(0, color='black', linewidth=0.8)
# Set custom grid intervals
x_grid = np.arange(-5, 6)
y_grid = np.arange(-5, 6)
plt.xticks(x_grid)
plt.yticks(y_grid)
plt.legend()
plt.show()
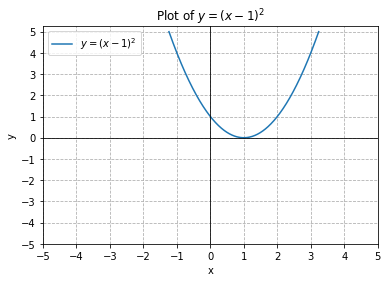
Example
From the plot above: 1. Evaluate
Do this by only looking at the graph and they try doing it algebraically.
Solution
To evaluate
, we find the input of on the horizontal axis. Moving up to the graph gives the point , giving an output of . So .To solve
, we find the value on the vertical axis because if then is the output. Moving horizontally across the graph gives two points with the output of : and . These give the two solutions to : or . This means and , or when the input is or , the output is .
[ ]: